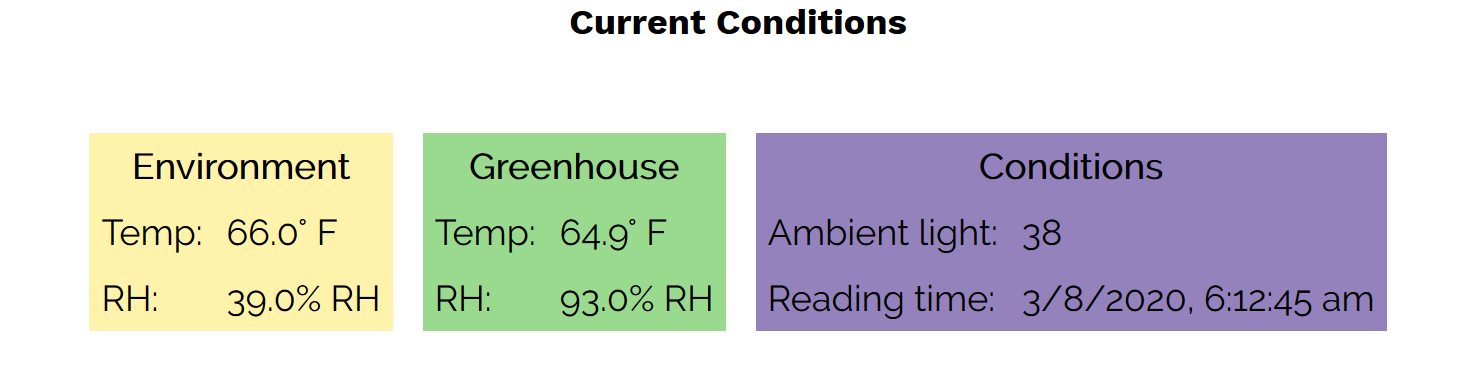
https
So, this morning I was surprised to wake up and discover my device had not updated in 3 hours. I tried to turn it off and on again, that didn't solve the problem, but at least the LEDs flickered on for half a second indicating it did have power and was likely working.
My first thought was that the website security certificate may have changed. So I followed up turning it off and on again with checking the /var/log/nginx/access.log which stated the following at 10:12:16 GMT
[08/Mar/2020:10:12:15 +0000] "POST /garden/api/readings.json/ HTTP/1.1" 201 113 "-" "ESP8266HTTPClient"
2600:1f16:269:da01:4e9f:c9d7:3ffe:6166 - - [08/Mar/2020:10:12:16 +0000] "GET /.well-known/acme-challenge/WrEvWgDp4cNpjjLpjglSja
1I90YFgAxTkW68YjoR2z4 HTTP/1.1" 200 87 "-" "Mozilla/5.0 (compatible; Let's Encrypt validation server; +https://www.letsencrypt.
org)"
2600:1f16:269:da01:4e9f:c9d7:3ffe:6166 - - [08/Mar/2020:10:12:16 +0000] "GET /.well-known/acme-challenge/ytSN6CxKtvhV19wks2bomt
q8ph6XdF6L7KjDA34aVso HTTP/1.1" 200 87 "-" "Mozilla/5.0 (compatible; Let's Encrypt validation server; +https://www.letsencrypt.
org)"
08/Mar/2020:10:12:15 +0000 was the time of my last successful update. I believe the next connection was from Let's Encrypt verifying that the update was successful.
I checked the certificate in my browser and it had this information at the bottom.
**Timestamp** 3/8/2020, 6:12:50 AM (Eastern Daylight Time)
**Signature Algorithm** SHA-256 ECDSA
I believe this was Let's Encrypt auto updating my certificate this morning. This is a month ahead of schedule, which reinforces that I don't know how that process works.
The issue is that the ESP2866 doesn't quite have the capability to connect to https servers. Or it does, but it requires a work around. I hard coded the SHA-1 fingerprint into the sketch. It wasn't ideal, it wasn't exactly what I wanted to do, but, I believed the certificate was good for another month, so it would work for the planned life of the project.
This morning, I will need to update the fingerprint on the sketch. I may change to using the root certificate which has a much longer life time. I may try to set a system up that auto updates the fingerprint.