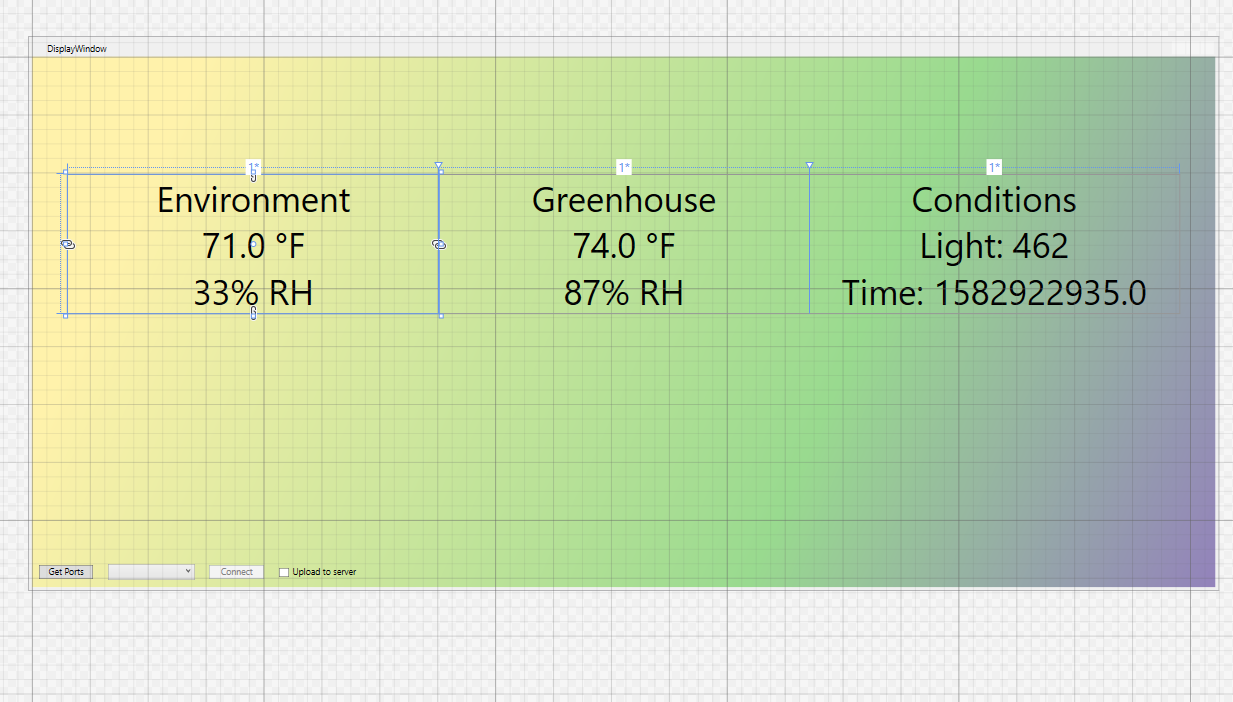
March 2, 2020, 7:56 p.m.
XAML
I started the C# of the project in a WinForms kind of place. The windows and code were tightly linked. I spent part of last week reworking the code to separate the project into a mvvm pattern. It's more work, but I find it very comforting that I can just delete a window and start over. I still need to create some converters to display the Unix Timestamp as a date. I would like to add charts in the next iteration.
<Window x:Class="Arduino_Greenhouse.View.DisplayWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:Arduino_Greenhouse.View"
xmlns:vm="clr-namespace:Arduino_Greenhouse.ViewModel"
mc:Ignorable="d"
Title="DisplayWindow"
WindowState="Maximized" d:DesignWidth="1642.688" d:DesignHeight="763.75"
>
<Window.Resources>
<vm:DisplayVM x:Key="vm"/>
</Window.Resources>
<Grid DataContext="{StaticResource vm}">
<Grid.RowDefinitions>
<RowDefinition Height="3*"/>
<RowDefinition Height="*"/>
<RowDefinition Height="Auto"/>
</Grid.RowDefinitions>
<Grid.Background>
<LinearGradientBrush EndPoint="0,0" StartPoint="1,1">
<GradientStop Color="#fff2ab" Offset="0.88" />
<GradientStop Color="#99da8f" Offset="0.33" />
<GradientStop Color="#9382bb" Offset="0" />
</LinearGradientBrush>
</Grid.Background>
<!--<Grid.ColumnDefinitions>
<ColumnDefinition/>
<ColumnDefinition/>
<ColumnDefinition/>
</Grid.ColumnDefinitions>-->
<Grid
Margin="50"
VerticalAlignment="Center"
HorizontalAlignment="Stretch"
>
<Grid.ColumnDefinitions>
<ColumnDefinition/>
<ColumnDefinition/>
<ColumnDefinition/>
</Grid.ColumnDefinitions>
<StackPanel Grid.Column="0" Width="Auto" >
<TextBlock Text="Environment" FontSize="48" TextAlignment="Center"/>
<TextBlock Text="{Binding sensorReading.temp1, StringFormat={}{0:f1} °F}" FontSize="48" TextAlignment="Center"/>
<TextBlock Text="{Binding sensorReading.rh1, StringFormat={}{0}% RH}" FontSize="48" TextAlignment="Center"/>
</StackPanel>
<StackPanel Grid.Column="1" Width="Auto">
<TextBlock Text="Greenhouse" FontSize="48" TextAlignment="Center"/>
<TextBlock Text="{Binding sensorReading.temp2, StringFormat={}{0:f1} °F}" FontSize="48" TextAlignment="Center" />
<TextBlock Text="{Binding sensorReading.rh2, StringFormat={}{0}% RH}" FontSize="48" TextAlignment="Center" />
</StackPanel>
<StackPanel Grid.Column="3" Grid.Row="0" Width="Auto">
<TextBlock Text="Conditions" FontSize="48" TextAlignment="Center"/>
<TextBlock Text="{Binding sensorReading.light, StringFormat=Light: {0}}" FontSize="48" TextAlignment="Center" />
<TextBlock Text="{Binding sensorReading.timestamp, StringFormat={}Time: {0:f1}}" FontSize="48" TextAlignment="Center"/>
</StackPanel>
</Grid>
<!--
Here lies the connection buttons
-->
<StackPanel Orientation="Horizontal" Grid.Row="2" DataContext="{StaticResource vm}">
<Button x:Name="btnGetPorts"
Content="Get Ports"
HorizontalAlignment="Left" Margin="10" Grid.Row="1" VerticalAlignment="Center" Width="75"
Command="{Binding GetComPortsCommand}"
/>
<ComboBox x:Name="cboPorts"
ItemsSource="{Binding ComPortList, NotifyOnSourceUpdated=True}"
SelectedIndex="0"
SelectedItem="{Binding SelectedComPort, UpdateSourceTrigger=PropertyChanged}"
HorizontalAlignment="Left" Margin="10" Grid.Row="1" VerticalAlignment="Center"
Width="120" RenderTransformOrigin="0.777,2.853"/>
<Button x:Name="btnConnect"
Command="{Binding ConnectToArduinoCommand}"
CommandParameter="{Binding SelectedComPort}"
Content="{Binding ConnectButtonContent, NotifyOnSourceUpdated=True}"
HorizontalAlignment="Left" Margin="10" Grid.Row="1" VerticalAlignment="Center" Width="75">
</Button>
<CheckBox x:Name="cbUpload" Content="Upload to server" HorizontalAlignment="Left" Margin="10" Grid.Row="1" VerticalAlignment="Center" />
</StackPanel>
</Grid>
</Window>